Authenticate O Series On-Premise and On-Demand
Learn about O Series API authentication for On-Premise and On-Demand deployments.
Invoke the O Series APIs
To be authenticated and authorized to invoke the Vertexยฎ O Series APIs for On-Demand and On-Premise deployments, you need to:
- Create a service account with the API User role.
- Add an API credential to generate a client ID and a client secret.
- Use your client ID and client secret to obtain an access token.
The access token can then be used to invoke the RESTful API.
Note:
See Authenticate O Series Cloud for details on authentication for Cloud deployments.
Create a service account
Note:
If you previously created a service account with the API User role for use with the O Series SOAP APIs, you can reuse that account if the configuration API permissions for the role are enabled.
- In O Series, select System > Security > Users.
- Click Add and create a service account.
- Assign the API User role to the service account.
- Click Save User.
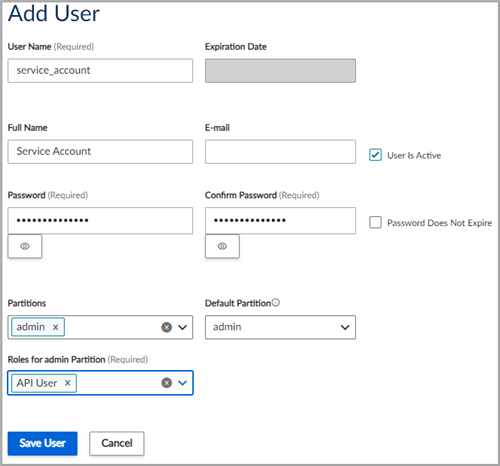
About the API User role
This role:
- Is required to invoke the RESTful API in O Series On-Premise and On-Demand environments
- Enables an assigned user to authorize communication between a connector external interface and the O Series web services
- Can be assigned by both the Master Administrator and the Partition System Administrator
- Does not provide access to features on the O Series user interface
You can create a user-defined role that has a subset of the API User role's permissions.
Generate a client ID and a client secret
To generate a client ID and client secret in O Series On-Premise and On-Demand, you need to add an API credential.
-
Select System > Security > API Credentials.
-
Click Add .
-
Enter your information for the following:
- User Name (This field is required.) - The service account you created with the API User role.
- Credential Issuer (This field is required.) - The entity that generates the credential. If you have configured an external IDP in O Series, that IDP can be selected. Otherwise, select the default option of O Series Internal - Open Id.
- Credential Expires in Days (This field is required.) - The API client credential life in number of days. The value must be an integer greater than or equal to 0. If the value is set to 0, the client credential does not expire.
- Token Expires in Seconds - The access token life in number of seconds. Shorter token lifetimes may minimize the possibility of the token being used maliciously. A token lifetime that is too short will require you to re-authenticate more frequently. The value must be a positive number and cannot be zero (
0
). If0
is entered, the value defaults to one second. If the field is left blank, the value defaults to 1800 seconds (30 minutes).
-
Click Save.
The API Credentials Confirmation dialog box displays the client ID and client secret.
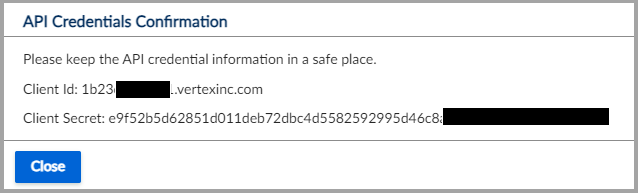
Cautions:
- The client secret is displayed only once. You must manually record the client secret when it is displayed. After you exit the dialog box, the client secret cannot be retrieved. If you lose the client secret, you can create a new client secret for the client ID.
- Vertex's authentication process rejects any access token request in which client credentials are provided in the URL as parameters.
Obtain an access token
Each call to the REST API requires a valid access token. By default, you can submit up to 10 access token requests to the standard access token endpoint every 8 hours. Exceeding the rate limit generates an error.
Note:
One access token can be used numerous times within its lifetime until it expires, at which point a new access token must be requested.
To help manage your Vertex RESTful API access tokens and reduce the need for frequent token generation, you can implement local token caching using the standard access token endpoint.
Token requests and responses
To obtain an access token with the standard token endpoint, submit a request to the appropriate endpoint URL by using an HTTP POST:
Vertex product | Endpoint URL |
---|---|
O Series On-Demand | https://<CUSTOMER>.ondemand.vertexinc.com/oseries-auth/oauth/token |
O Series On-Premise | https://<SERVER:PORT>/oseries-auth/oauth/token |
For example:
- O Series On-Demand:
https://examplecustomer.ondemand.vertexinc.com/oseries-auth/oauth/token
- O Series On-Premise:
https://MyServer:1234/oseries-auth/oauth/token
Provide these parameters in the request:
Parameter name | Field location | Definition | Type |
---|---|---|---|
client_id | In the request body or in the Authorization header | The client ID provided by Vertex for the custom integration | String, required |
client_secret | In the request body or in the Authorization header | The client secret provided by Vertex for the custom integration | String, required |
grant_type | In the request body | The string client_credentials | String, required |
The client_id
and client_secret
parameters are issued for each integration against the RESTful API. Because they are confidential values, do not expose them to any users of the integration.
The response from the call to the token endpoint is a JSON object.
Security recommendation
Verify that your implementation is passing your client credentials in the API request body or the Authorization header, and not in the URL parameters when requesting bearer tokens.
As of the SR17 MP1 O Series release, Vertex's authentication process rejects any access token request in which client credentials are provided in the URL as parameters.
Sample token requests
The client_id
and client_secret
can be passed in the body of the request or in the request header.
Sample requests with information in request body
Here are access token requests submitted using cURL with all required information in the request body and a content type of application/json
:
curl --location 'http://MyServer:1234/oseries-auth/oauth/token'
--header 'content-type: application/json'
--data '{
"client_id": "<your-client-id>",
"audience": "<audience>",
"client_secret": "<your-client-secret>",
"grant_type": "client_credentials"
}'
curl --location 'http://examplecustomer.ondemand.vertexinc.com/oseries-auth/oauth/token'
--header 'content-type: application/json'
--data '{
"client_id": "<your-client-id>",
"client_secret": "<your-client-secret>",
"audience": "<audience>",
"grant_type": "client_credentials"
}'
Here are sample requests with all required information in the request body with a content type of application/x-www-form-urlencoded
:
curl --location 'http://MyServer:1234/oseries-auth/oauth/token'
--header 'Content-Type: application/x-www-form-urlencoded'
--data-urlencode 'client_id=<your-client-id>'
--data-urlencode 'client_secret=<your-client-secret>'
--data-urlencode 'audience=<audience>'
--data-urlencode 'grant_type=client_credentials'
curl --location 'http://examplecustomer.ondemand.vertexinc.com/oseries-auth/oauth/token'
--header 'Content-Type: application/x-www-form-urlencoded'
--data-urlencode 'client_id=<your-client-id>'
--data-urlencode 'client_secret=<your-client-secret>'
--data-urlencode 'audience=<audience>'
--data-urlencode 'grant_type=client_credentials'
An access token request submitted using C# with information in the request body may look like the following:
var client = new RestClient("http://{base_uri}/oseries-auth/oauth/token");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("application/x-www-form-urlencoded", "grant_type=client_credentials&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&audience=YOUR_API_IDENTIFIER", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Sample requests with information in Authorization header
When passing the client_id
and client_secret
in the request header, you need to set the Authorization header key and value to:
Bearer {base64(client_id:client_secret)}
Here is a sample request passing the client_id
and client_secret
in the Authorization header with a content type of application/json
:
curl --location 'http://{base_uri}/oseries-auth/oauth/token'
--header 'content-type: application/json'
--header 'Authorization: Basic base64_encode(<your-client-id>:<your-client-secret>)'
--data '{
"audience": "<audience>",
"grant_type": "client_credentials"
}'
Here is a sample request passing the client_id
and client_secret
in the Authorization header with a content type of application/x-www-form-urlencoded
:
curl --location 'http://{base_uri}/oseries-auth/oauth/token'
--header 'Content-Type: application/x-www-form-urlencoded'
--header 'Authorization: Basic base64_encode(<your-client-id>:<your-client-secret>)'
--data-urlencode 'grant_type=client_credentials'
Sample token responses
After submitting a request to the endpoint URL, the API responds with a success response or an error response.
Success response example
If the call is successful (HTTP status code = 200), the JSON object has multiple properties. For example:
{
"access_token": "valid_token_ID",
"token_type": "Bearer",
"expires_in": 3599,
"scope": "profile"
}
The access_token
and token_type
parameters are needed to make calls against the Vertex RESTful API. The returned expires_in
parameter defines the number of seconds until the access token expires. After this time, a new token must be requested. The scope
parameter is for informational use only.
Error response
If an error occurs (HTTP status code != 200), the JSON object has one "error" property with a message that describes the reason for failure. For example:
{
"error" : "invalid_client"
}
Authorize a request
All API requests against the RESTful API endpoints must be made over HTTPS. When making a request, set the access token in the Authorization header of the request with the token type, and access token or access token variable. For example:
Authorization: Bearer Token {ACCESS_TOKEN or ACCESS_TOKEN_VARIABLE}
Or, use the above ACCESS_TOKEN_VARIABLE
request to send a request via Postman:
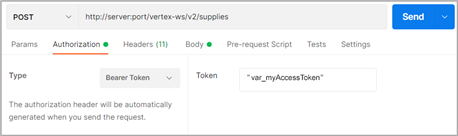
Caution:
This example is for illustration purposes only. It will not work in the API.
Updated 3 months ago