Authenticate O Series Cloud
Learn about API authentication for O Series Cloud deployments.
Invoke the O Series APIs
If you are using a Vertexยฎ O Series RESTful API to integrate with your financial host system with O Series Cloud, follow these procedures to invoke the O Series API.
To be authenticated and authorized to invoke the O Series APIs for Cloud deployments, you need to:
- Create an API credential to generate a client ID and a client secret.
- Use the client ID and client secret to obtain an access token.
- (Optional) Configure token caching.
The access token can then be used to invoke the RESTful API.
Note:
See Authenticate O Series On-Premise and On-Demand for details on authentication for On-Premise and On-Demand deployments.
Create an API credential in O Series Cloud
Note:
You must have the Cloud Partition System Administrator role to create an API credential.
-
Click Account Settings, the gear wheel icon on the contextual bar of any page.
-
Select Security & Credentials.
-
Click Create a Credential.
-
(Required) Select the API this credential will map to from the drop-down list.
- For calculation and configuration APIs, select Rest API for O Series Calculation.
- For reconciliation report APIs, select Vertex Public REST API.
-
(Required) Select the Partition this credential will map to from the drop-down list.
-
(Optional) Enter a Credential Description.
-
(Required) Select an identity provider (IDP). VERX IDP is the Vertex-recommended Open Authorization (OAuth) IDP.
Note:
- VERX IDP is not currently available for O Series On-Premise or On-Demand deployments. If you are also integrating with O Series On-Premise or On-Demand, your integration must account for different IDP options per deployment.
- The Legacy IDP is deprecated and will be shut down in September 2025. If you are currently using the Legacy IDP, you need to start using the VERX IDP before this date. When the Legacy IDP is shut down, it will no longer create credentials. Instructions for switching to the VERX IDP from the Legacy IDP are available in Change IDPs in O Series Cloud.
-
Click Save the Credential.The New Client Credential Created dialog box displays the client ID and client secret.
Caution:
The client secret is displayed only once. You must manually record the client secret when it is displayed. After you exit the dialog box, the client secret cannot be retrieved. If you lose the client secret, you can create a new client secret for the client ID.
-
Allow 15 minutes for processing before using your client ID and client secret to obtain an access token.
OAuth for O Series Cloud
VERX IDP is the Vertex-recommended Open Authorization (OAuth) identity provider (IDP) available in O Series Cloud. Select VERX IDP as the IDP when creating an API credential to take advantage of OAuth capabilities, including the Vertex token caching service.
OAuth token limits
By default, you can submit up to 10 access token requests to the standard OAuth access token endpoint every 8 hours.
Change IDPs in O Series Cloud
API credentials in O Series Cloud cannot be modified. To assign a different IDP for your API credential, create a new credential:
- Create a new credential and select the new identity provider. Your new credential generates a new client ID and a new client secret.
- Use the new ID and secret to obtain a new access token.
Audience parameter required
To obtain an access token from an OAuth identity provider, the audience
parameter is required in the body of your authorization request .
Obtain an access token
Each call to the REST API requires a valid access token. By default, you can submit up to 10 access token requests to the standard access token endpoint every 8 hours. Exceeding the rate limit generates an error.
Note:
One access token can be used numerous times within its lifetime until it expires, at which point a new access token must be requested.
To help manage your Vertex RESTful API access tokens and reduce the need for frequent token generation, you can implement local token caching using the standard access token endpoint.
For O Series Cloud deployments, the Vertex token caching service is available for the VERX IDP OAuth IDP.
Local token caching
To obtain an access token, submit a request to the appropriate endpoint URL by using an HTTP POST:
Vertex product | Endpoint URL |
---|---|
O Series Cloud | https://auth.vertexsmb.com/identity/connect/token |
Provide these parameters in the request body:
Parameter name | Field location | Definition | Type |
---|---|---|---|
audience | In the request body | โ ๏ธThis parameter is required for the VERX IDP (OAuth) identity provider . The audience for the token, which is the base URI for the API. The value depends on the API you are calling. | String, required for VERX IDP |
client_id | In the request body or in the Authorization header | The client ID provided by Vertex for the custom integration | String, required |
client_secret | In the request body or in the Authorization header | The client secret provided by Vertex for the custom integration | String, required |
grant_type | In the request body | The string client_credentials | String, required |
Audience parameter required for OAuth IDP
To obtain an access token from an OAuth identity provider, the audience
parameter is required in the body of your authorization request. This parameter identifies the API for which the token grants access.
When requesting a token for an O Series Cloud API credential with VERX IDP selected as the identity provider, use the base URI for the API you are calling as the audience
value in your token request.
For example, the audience
parameter for the Tax Calculation and Tax GIS API and the Tax Configuration API is:
'audience= https://calcconnect.vertexsmb.com'
Security recommendation
Verify that your implementation is passing your client credentials in the API request body or the Authorization header, and not in the URL parameters when requesting bearer tokens.
As of the May 2024 O Series release, Vertex's authentication process rejects any access token request in which client credentials are provided in the URL as parameters.
Sample token requests
The client_id
and client_secret
can be passed in the body of the request or in the request header.
Sample requests with information in request body
Here is an access token request submitted using cURL with all required information in the request body and a content type of application/json
:
curl --location '<https://auth.vertexsmb.com/identity/connect/token>'
--header 'content-type: application/json'
--data '{
"client_id": "<your-client-id>",
"client_secret": "<your-client-secret>",
"audience": "<audience>",
"grant_type": "client_credentials"
}'
Here is a sample request with all required information in the request body with a content type of application/x-www-form-urlencoded
:
curl --location 'https://auth.vertexsmb.com/identity/connect/token'
--header 'Content-Type: application/x-www-form-urlencoded'
--data-urlencode 'client_id=<your-client-id>'
--data-urlencode 'client_secret=<your-client-secret>'
--data-urlencode 'audience=<audience>'
--data-urlencode 'grant_type=client_credentials'
An access token request submitted using C# with information in the request body may look like the following:
var client = new RestClient("https://auth.vertexsmb.com/identity/connect/token");
var request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
request.AddParameter("application/x-www-form-urlencoded", "grant_type=client_credentials&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&audience=YOUR_API_IDENTIFIER", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Sample requests with information in Authorization header
When passing the client_id
and client_secret
in the request header, you need to set the Authorization header key and value to:
Bearer {base64(client_id:client_secret)}
Here is a sample request passing the client_id
and client_secret
in the Authorization header with a content type of application/json
:
curl --location 'https://auth.vertexsmb.com/identity/connect/token'
--header 'content-type: application/json'
--header 'Authorization: Basic base64_encode(<your-client-id>:<your-client-secret>)'
--data '{
"audience": "<audience>",
"grant_type": "client_credentials"
}'
Here is a sample request passing the client_id
and client_secret
in the Authorization header with a content type of application/x-www-form-urlencoded
:
curl --location 'https://auth.vertexsmb.com/identity/connect/token'
--header 'Content-Type: application/x-www-form-urlencoded'
--header 'Authorization: Basic base64_encode(<your-client-id>:<your-client-secret>)'
--data-urlencode 'audience=<audience>'
--data-urlencode 'grant_type=client_credentials'
Sample token responses
After submitting a request to the endpoint URL, the API responds with a success response or an error response.
Success response example
If the call is successful (HTTP status code = 200), the JSON object has multiple properties. For example:
{
"access_token": "valid_token_ID",
"token_type": "Bearer",
"expires_in": 3599,
"scope": "profile"
}
The access_token
and token_type
parameters are needed to make calls against the Vertex RESTful API. The returned expires_in
parameter defines the number of seconds until the access token expires. After this time, a new token must be requested. The scope
parameter is for informational use only.
Error response example
If an error occurs (HTTP status code != 200), the JSON object has one "error" property with a message that describes the reason for failure. For example:
{
"error" : "invalid_client"
}
Authorize a request
All API requests against the RESTful API endpoints must be made over HTTPS. When making a request, set the access token in the Authorization header of the request with the token type, and access token or access token variable. For example:
Authorization: Bearer Token {ACCESS_TOKEN or ACCESS_TOKEN_VARIABLE}
Or, use the above ACCESS_TOKEN_VARIABLE
request to send a request via Postman:
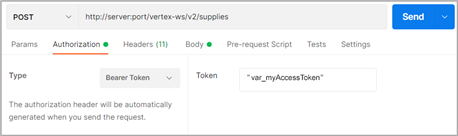
Caution:
This example is for illustration purposes only. It will not work in the API.
Updated 3 months ago